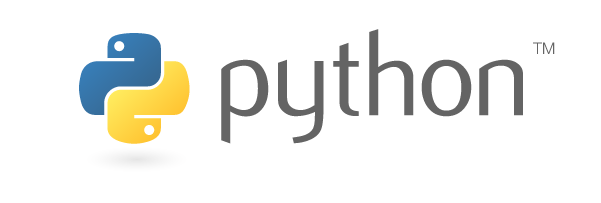
Python has multiple ways to format text strings. The most common being %-formatting
and str.format()
. These existing ways of formatting are either error prone, inflexible, or cumbersome. And then PEP 498 introduced the f-strings also known as Literal String Interpolation.
PEP 498 now gives us a very simple and clear way of formatting strings. f-String is a more readable and concise way of formatting python strings. It is also less prone to error. But it is important to remember that these are available after Python 3.6 only.
f-strings are string literals start with f
at the beginning and have the expressions inside curly braces which will be replaced with their values.
blog_name = 'OddBlogger' print(f'You are reading this blog on {blog_name}') # Output # You are reading this blog on OddBlogger
f-Strings are expressions that are manipulated at runtime
It means f-Strings provide you the capability to embed expressions inside string literals and they will be evaluated at runtime like any other python expression. And they are formatted using the __format__
protocol. So the following works absolutely fine:
print(f"2 times 2 is {2*2}") # Outputs: 2 times 2 is 4 def transform(name): name.capitalize() name = "oddblogger" print(f"You are reading this on {transform(name)}") # Outputs: You are reading this on Oddblogger
The existing string formatting mechanisms are not deprecated. Yet.
Yes. The “older” ways of formatting strings in Python are not yet deprecated. So you may still use those especially if you are working with a Python version of < 3.6.
f-Strings are faster.
f-Strings are faster than both, %-formatting
and str.format()
. How much you may ask? Here is the difference:
import timeit timeit.timeit("""blog_name="OddBlogger" 'You are reading this on {}'.format(blog_name)""",number=10000) timeit.timeit("""blog_name="OddBlogger" 'You are reading this on %s' % (blog_name)""",number=10000) timeit.timeit("""blog_name="OddBlogger" 'You are reading this on {blog_name}'""",number=10000) # Output # 0.0035877509999409085 # 0.0028783220000150322 # 0.0001674219997767068
You can do much more with f-String like multiline strings, strings with dictionary expressions, with special characters etc. For more details head over to the the official developer guide – PEP 498.