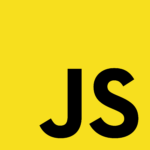
Javascript is a single-threaded language yet it works on a concurrency model with the help of the javascript event loop. Javascript event loop is responsible for the execution of the code, collection. It takes care of the processing of the events as well as callbacks and the execution of all functions lying inside the call stack as well as callback queue.
Let’s study more about these concepts below to understand the role of event loop in javascript more deeply.
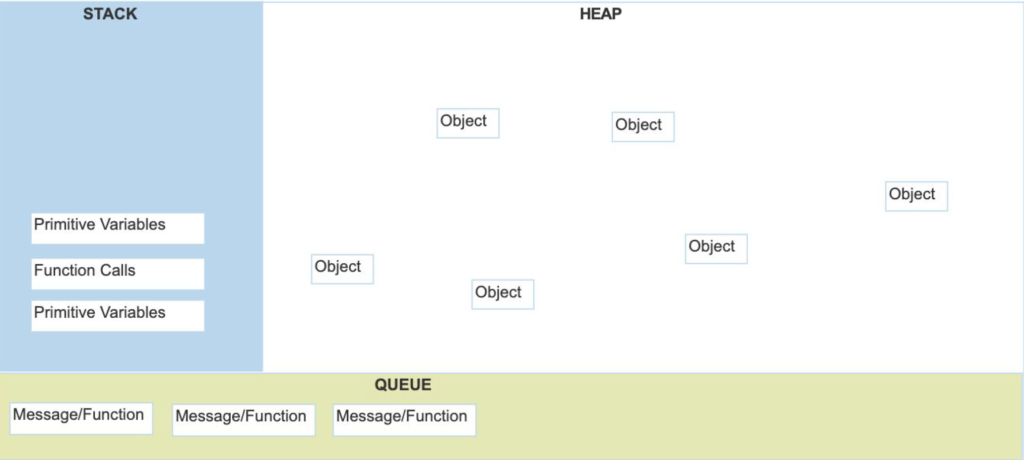
Call Stack
The call stack is used for storing function calls. And as the name suggests, it follows the LIFO order.
It is basically responsible for keeping the track of all the operations of the code. A function call gets popped from the stack once, its execution is finished.
Heap
Heap is used for storing objects.
Queue
Queues are used to store messages which also have an associated function. It follows the FIFO order and as the message gets selected for execution, it’s corresponding function gets called.
Javascript Event Loop
The event loop plays a very important part in the single-threaded javascript.
It handles the asynchronous calls, non-blocking I/O effectively in order to optimize the performance of javascript. Non-blocking nature of javascript is achieved by events and callbacks.
For example:-
setTimeout (function callback() { console.log('Message from a callback function'); }, 0);
The event loop constantly checks the call stack for any function calls and also adds any function found during execution on top of the stack.
Whenever an async function is called, it is sent to a browser API. These are APIs built into the browser. Based on the command received from the call stack, the API starts its own single-threaded operation, and once it completes the execution, the function is sent to the callback queue.
If the call stack is empty, it looks into the callback queue and gets the first message/function from the callback queue. This kind of iteration is called a ‘tick’ in the event loop.
Let us understand the javascript event loop using this example-
function first() { console.log(‘first’); } function second() { console.log(‘second’); } function third() { console.log(‘third’); } first(); setTimeout(second, 100); // Invoke `second` after 100ms third();
Output
first
third
second
Steps
first()
will push on top of the call stack.- While executing first, we come across
console.log(‘first’)
which goes inside the call stack. console.log(‘first’)
will pop and ‘first’ gets print on the console.first()
will pop.setTimeout(second, 0)
will push on top of the call stack.second()
will push to the web APIs which wait for 100ms before pushing it to the callback queue.setTimeout(second, 0)
will pop.third()
will push on top of the call stack.second()
will push to the callback queue.- While executing third, we come across
console.log(‘third’)
which goes inside the call stack. console.log(‘third’)
will pop and ‘third’ will print on the console.third()
will pop.second()
will pop from the callback queue and will push on top of the call stack.- While executing second, we come across
console.log(‘second’)
which goes inside the call stack. console.log(‘second’)
will pop and ‘second’ will print on the console.second()
will pop.
Click here to view the visualization of the event loop model for the above example.
Conclusion
The event loop acts as a binding loop between the code, call stack, callback queue, and browser APIs. It constantly checks the call stack for any new function calls. And if there are no new function calls, it will check the callback queue to check if any function call is waiting to be pushed to the call stack. Hence, it serves as an integral part of the javascript ecosystem.
Thanks for reading. Share your thoughts in comments.